What is DART API?
Dart api provided the ability to external system to connect with the Dart.By using api, user can create and manage the dart's users externally.In here bearer token use to identify the api users.
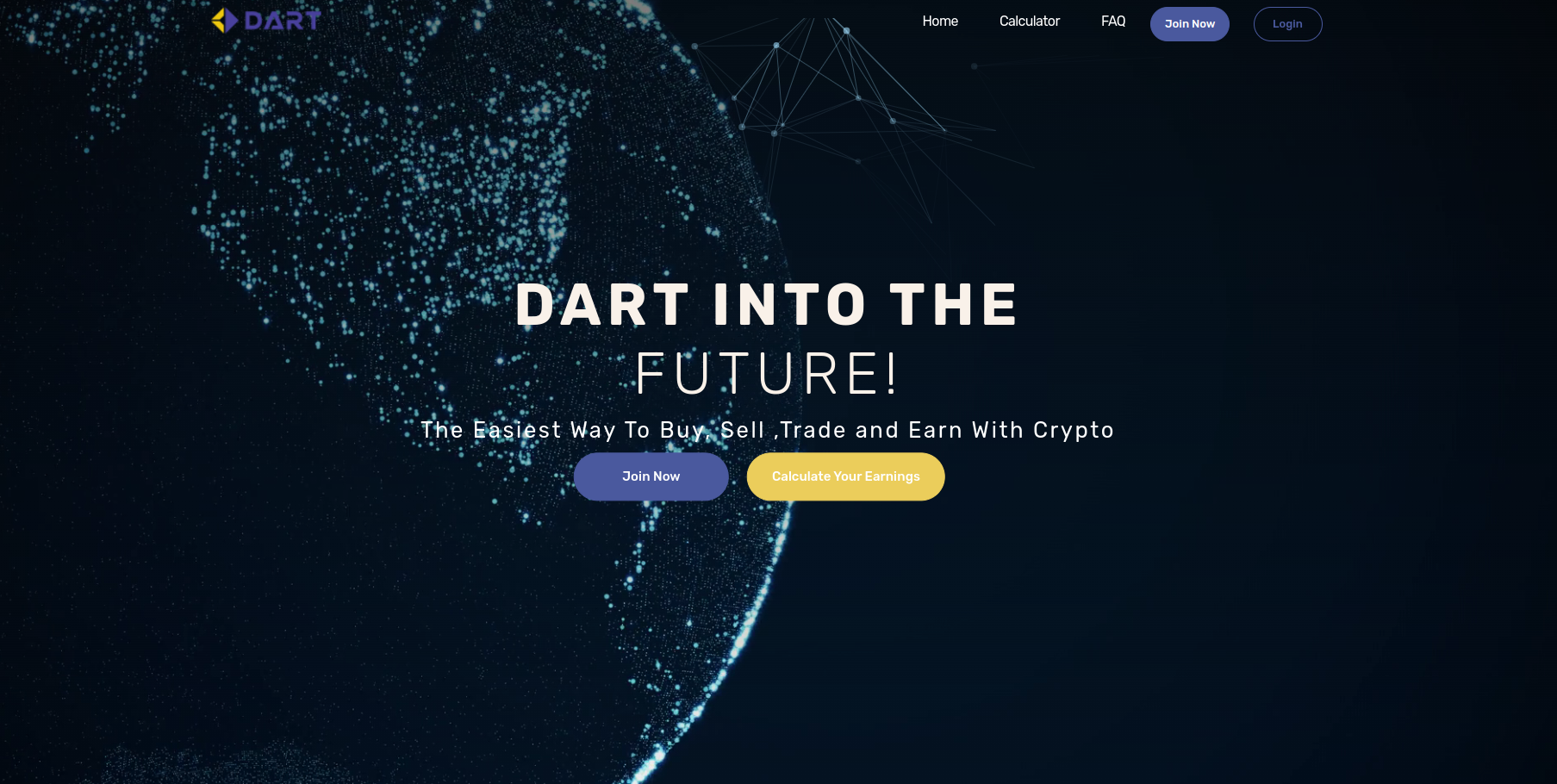
API Url: https://api.dart.cash/api
Dart api provided the ability to external system to connect with the Dart.By using api, user can create and manage the dart's users externally.In here bearer token use to identify the api users.
API Url: https://api.dart.cash/api
As below after create API token using for authenticate with the API, simply append the bearer token GET parameter to the API's base URL:
1$request->setHeader(array(2 'Authorization' => 'Bearer <token>'3));
In order to get customer details an HTTP GET request containing a series of parameters and objects to the API's customer/get endpoint is required. All parameters and objects are specified below:
Name | Description |
---|---|
dart_user_name | This parameter is used in order to specify a customer user name. |
1$request = new HTTP_Request2(); 2$request->setUrl('https://api.dart.cash/api/customer/get?dart_user_name=billyjoel'); 3$request->setMethod(HTTP_Request2::METHOD_GET); 4$request->setConfig(array( 5 'follow_redirects' => TRUE 6)); 7$request->setHeader(array( 8 'Accept' => 'application/json', 9 'Authorization' => 'Bearer 2|BtyCiKlUQ8wSDlhCAXYVMljlFudQKb5AqWhLOmTT'10));
1{2 "first_name": "Billy",3 "last_name": "Joel",4 "dart_user_name": "billyjoel",5 "email": "billy@gmail.com"6}
In order to create external customer an HTTP POST request containing a series of parameters and objects to the API's customer/create endpoint is required. All parameters and objects are specified below:
Name | Description |
---|---|
first_name | This parameter is used in order to specify a customer first name. |
last_name | This parameter is used in order to specify a customer last name. |
user_name | This parameter is used in order to specify a customer user name. |
This parameter is used in order to specify a customer email. | |
password | This parameter is used in order to specify a customer password. |
1$request = new HTTP_Request2(); 2$request->setUrl('https://api.dart.cash/api/customer/create'); 3$request->setMethod(HTTP_Request2::METHOD_POST); 4$request->setConfig(array( 5 'follow_redirects' => TRUE 6)); 7$request->setHeader(array( 8 'Accept' => 'application/json', 9 'Authorization' => 'Bearer 2|BtyCiKlUQ8wSDlhCAXYVMljlFudQKb5AqWhLOmTT'10));11$request->addPostParameter(array(12 'first_name' => 'Billy ',13 'last_name' => 'Joel',14 'user_name' => 'billyjoel',15 'email' => 'billy@gmail.com',16 'password' => 'billy@123#'17));
1"Customer Created Successfully."
In order to edit external customer an HTTP POST request containing a series of parameters and objects to the API's customer/update endpoint is required. All parameters and objects are specified below:
Name | Description |
---|---|
dart_user_name | This parameter is used in order to specify a customer user name. |
first_name | This parameter is used in order to specify a customer first name. |
last_name | This parameter is used in order to specify a customer last name. |
This parameter is used in order to specify a customer email. | |
password | This parameter is used in order to specify a customer password. |
1$request = new HTTP_Request2(); 2$request->setUrl('https://api.dart.cash/api/customer/update'); 3$request->setMethod(HTTP_Request2::METHOD_POST); 4$request->setConfig(array( 5 'follow_redirects' => TRUE 6)); 7$request->setHeader(array( 8 'Accept' => 'application/json', 9 'Authorization' => 'Bearer 2|BtyCiKlUQ8wSDlhCAXYVMljlFudQKb5AqWhLOmTT'10));11$request->addPostParameter(array(12 'dart_user_name' => 'billyjoel',13 'first_name' => 'Billy',14 'last_name' => 'Joel',15 'email' => 'billy@gmail.com',16 'password' => 'billy@123'17));
1"Customer Updated Successfully."
In order to restrict for trade scripts an HTTP POST request containing a series of parameters and objects to the API's customer/update-tsp-status endpoint is required. All parameters and objects are specified below:
Name | Description |
---|---|
dart_user_name | This parameter is used in order to specify a customer user name. |
external_tsp_status | Set to false in order to restrict for trade scripts. |
tsp_duration | Set an integer as number of hours to deactivate trade scripts. |
1$request = new HTTP_Request2(); 2$request->setUrl('https://api.dart.cash/api/customer/update-tsp-status'); 3$request->setMethod(HTTP_Request2::METHOD_POST); 4$request->setConfig(array( 5 'follow_redirects' => TRUE 6)); 7$request->setHeader(array( 8 'Accept' => 'application/json', 9 'Authorization' => 'Bearer 2|BtyCiKlUQ8wSDlhCAXYVMljlFudQKb5AqWhLOmTT'10));11$request->addPostParameter(array(12 'dart_user_name' => 'billyjoel',13 'external_tsp_status' => 'false',14 'tsp_duration' => '10',15));
1"Customer Tsp Access Disabled"
In order to get customer trade script details an HTTP GET request containing a series of parameters and objects to the API's customer/trade-script-details endpoint is required. All parameters and objects are specified below:
Name | Description |
---|---|
dart_user_name | This parameter is used in order to specify a customer user name. |
1$request = new HTTP_Request2(); 2$request->setUrl('https://api.dart.cash/api/customer/trade-script-details?dart_user_name=billyjoel'); 3$request->setMethod(HTTP_Request2::METHOD_GET); 4$request->setConfig(array( 5 'follow_redirects' => TRUE 6)); 7$request->setHeader(array( 8 'Accept' => 'application/json', 9 'Authorization' => 'Bearer 2|BtyCiKlUQ8wSDlhCAXYVMljlFudQKb5AqWhLOmTT'10));
1{ 2"data": [ 3{ 4"trade_script_name": "Full Velocity Members Only", 5"initial_amount": "49.25", 6"current_amount": "49.2500", 7"status": 5, 8"total_deposit": "50.0000", 9"activate_date": "2022-06-08"10}11]12}
In order to get customer total balance of USDC an HTTP GET request containing a series of parameters and objects to the API's customer/usdc-balance endpoint is required. All parameters and objects are specified below:
Name | Description |
---|---|
dart_user_name | This parameter is used in order to specify a customer user name. |
1$request = new HTTP_Request2(); 2$request->setUrl('https://api.dart.cash/api/customer/usdc-balance?dart_user_name=billyjoel'); 3$request->setMethod(HTTP_Request2::METHOD_GET); 4$request->setConfig(array( 5 'follow_redirects' => TRUE 6)); 7$request->setHeader(array( 8 'Accept' => 'application/json', 9 'Authorization' => 'Bearer 2|BtyCiKlUQ8wSDlhCAXYVMljlFudQKb5AqWhLOmTT'10));
1{2 "total_usdc_balance":"980.04"3}
In order to get customer custom token value an HTTP GET request containing a series of parameters and objects to the API's customer/token-value endpoint is required. All parameters and objects are specified below:
Name | Description |
---|---|
dart_user_name | This parameter is used in order to specify a customer user name. |
token | This parameter is used in order to specify a token name. |
1$request = new HTTP_Request2(); 2$request->setUrl('https://api.dart.cash/api/customer/token-value?dart_user_name=billyjoel&token=Chaos'); 3$request->setMethod(HTTP_Request2::METHOD_GET); 4$request->setConfig(array( 5 'follow_redirects' => TRUE 6)); 7$request->setHeader(array( 8 'Accept' => 'application/json', 9 'Authorization' => 'Bearer 2|BtyCiKlUQ8wSDlhCAXYVMljlFudQKb5AqWhLOmTT'10));
1{2 "token_value":"19.96"3}
In order to transfer an amount to a customer's main wallet. post an HTTP POST request containing a series of parameters and objects to the API's customer/transfer-amount endpoint is required. All parameters and objects are specified below:
1$request = new HTTP_Request2(); 2$request->setUrl('https://api.dart.cash/api/customer/transfer-amount'); 3$request->setMethod(HTTP_Request2::METHOD_POST); 4$request->setConfig(array( 5 'follow_redirects' => TRUE 6)); 7$request->setHeader(array( 8 'Accept' => 'application/json', 9 'Authorization' => 'Bearer 2|BtyCiKlUQ8wSDlhCAXYVMljlFudQKb5AqWhLOmTT'10));
Name | Description |
---|---|
userId | This parameter is used in order to specify a customer user name. |
amount | This parameter is used in order to specify an amount. |
1{2 "userId" : "customer user name.",3 "amount" : "the amount api user wants to transfer to customer",4}
After activating your customer from the Dart system you will receive a Webhook HTTP POST response from this your-domain/webhook/customer-activate endpoint.
1{2 "message" : "External customer activated.",3 "old_dart_user_name" : "billyjoel",4 "new_dart_user_name" : "billyjoel"5}
After updating your customer details from the Dart system you will receive a Webhook HTTP POST response from this your-domain/webhook/customer-update endpoint.
1{2 "message" : "External customer information updated",3 "old_dart_user_name" : "billyjoel",4 "new_dart_user_name" : "billy"5}
After deactivating your customer from the Dart system you will receive a Webhook HTTP POST response from this your-domain/webhook/customer-deactivate endpoint.
1{2 "message" : "External customer deactivated."3 "old_dart_user_name" : "billyjoel",4 "new_dart_user_name" : "billyjoel"5}
After customer withdrawal or exit from the Dart system trade script you will receive a Webhook HTTP POST response from this your-domain/webhook/trade-script-withdrawal endpoint.
1{2 "message" : "External customer exit from trade script."3 "old_dart_user_name" : "billyjoel",4 "new_dart_user_name" : "billyjoel",5 "amount" => "2.00",6 "trade_script_name" => "Full Velocity Members Only"7}
1{2 "message" : "External customer withdraw from trade script."3 "old_dart_user_name" : "billyjoel",4 "new_dart_user_name" : "billyjoel",5 "amount" => "50.00",6 "trade_script_name" => "Full Velocity Members Only"7}